SpiderAdmin was introduced in Lesson 3 of this tutorial and explained in detail in Lesson 14 as a powerful tool for monitoring and administering a service mesh without adding a single line of code. The features we presented included data communication monitoring, configuration, log system exploration and some general maintenance aspects. In this lesson we are going to learn how, in very few lines of code, the tool can be extended to cover the logics of specific applications.
Our test case will be, what else, the Chat application that we have been dealing with along this tutorial. We will add the following elements to its SpiderAdmin page:
- Two extra columns in the Server Information table that show the current user name and the current room the user is chatting in (if any).
- An extra table – Room Activity – that shows the activity of all the chatters in the room.
- A “Ban Room” button that the system administrator can use to kick out and ban the user from the room.
Before dealing with the required modifications to the Chat
application, let’s put a small change in the ChatApp
class of the objectLib
package:
package org.spiderwiz.tutorial.objectLib; import org.spiderwiz.annotation.WizField; import org.spiderwiz.core.DataObject; /** * A DataObject class that represents a chat application within a specific chat room. It is the parent of all the chatters that * are in that application and joined the room that is the parent of this object. */ public class ChatApp extends DataObject { /** * Mandatory public static field for all data objects. */ public final static String ObjectCode = "CHTAPP"; @WizField private boolean banned = false; public boolean isBanned() { return banned; } public void setBanned(boolean banned) { this.banned = banned; } @Override protected String getParentCode() { return ChatRoom.ObjectCode; } @Override protected boolean isDisposable() { return false; } }
We add a property – a boolean banned
field (line 16) – that is set to true
when the application instance (identified by its UUID) is
banned from the parent room. We will see below how it works.
Back to the Chat application, here is ChatMain
:
package org.spiderwiz.tutorial.lesson18.chat; import java.io.PrintStream; import java.text.ParseException; import java.util.Collection; import java.util.Collections; import java.util.List; import org.spiderwiz.admin.data.OpResults; import org.spiderwiz.admin.data.PageInfo; import org.spiderwiz.admin.data.PageInfo.TableInfo.Style; import org.spiderwiz.admin.data.TableData; import org.spiderwiz.core.CatchAllFilter; import org.spiderwiz.core.DataObject; import org.spiderwiz.tutorial.objectLib.ActiveUserQuery; import org.spiderwiz.tutorial.objectLib.Chat; import org.spiderwiz.tutorial.objectLib.ChatApp; import org.spiderwiz.tutorial.objectLib.ChatHistoryQuery; import org.spiderwiz.tutorial.objectLib.ChatMessage; import org.spiderwiz.tutorial.objectLib.ChatRoom; import org.spiderwiz.tutorial.objectLib.Chatter; import org.spiderwiz.tutorial.objectLib.ConsoleMain; import org.spiderwiz.tutorial.objectLib.LoginQuery; import org.spiderwiz.zutils.ZDate; /** * Provides the entry point of the application. Initializes and executes the Spiderwiz framework. */ public class ChatMain extends ConsoleMain{ private static final String ROOT_DIRECTORY = ""; private static final String APP_NAME = "Chat Room Client"; private static final String APP_VERSION = "Z18.01"; // Version Z18.01: Lesson 18 modifications private static final String JOIN = "!join"; // join a chat room command private static final String LEAVE = "!leave"; // leave current chat room command private static final String LOGIN = "!login"; // start login procedure private static final String LOGOUT = "!logout"; // log the user out private static final String REGISTER = "!register"; // start registration procedure private static final String HISTORY = "!history"; // print my chat history private String myName = null; // The user login name private ZDate birthday = null; // User birth date. Get it when logging in private ChatterConsume chatter = null; // A Chatter object representing the user chatting in a specific room private ChatMessage message = null; // A ChatMessage object for committing chat messages private Chat privateMessage = null; // A Chat object for committing private messages /** * Class constructor with constant parameters. * @param confFileName configuration file name, provided as a command argument */ public ChatMain(String confFileName) { super(ROOT_DIRECTORY, confFileName, APP_NAME, APP_VERSION); } /** * @return the class instance as ChatMain type. */ public static ChatMain getInstance() { return (ChatMain)ConsoleMain.getInstance(); } /** * Application entry point. Instantiate the class and initialize the instance. * * @param args the command line arguments. The first argument is used as the configuration file name. */ /** * @param args the command line arguments */ public static void main(String[] args) { // Don't do anything if there is no configuration file name if (args.length == 0) { System.out.println("Configuration file has not been defined"); return; } new ChatMain(args[0]).init(); } /** * Print user instructions to the console. * @return true */ @Override protected boolean preStart() { System.out.printf( "Welcome to the dynamic chat system.n" + "To log in type !login.n" + "To log out type !logout.n" + "To register type !register.n" + "To join a chat room or change your current chat room, type "!join <room name>".n" + "To leave your chat room, type "!leave".n" + "To send a message in the current chat room just type the message " + "(a message cannot start with either '>' or '!').n" + "To send a private message type '>' followed by name of the user you want to send " + "the private message to, followed by ':' for by the message.n" + "To print the history of your own messages, type "!history <hours>h" or "!history <days>d"n" + "(for instance "!history 3h" to see messages since 3 hours ago and "!history 1d" to see messages since 1 day" + " ago).n" + "or type just "!history" to see all your messages.n" + "To exit type 'exit'.n" ); return true; } /** * @return the list of produced objects - Chatter, Chat, ChatMessage and LoginQuery */ @Override protected String[] getProducedObjects() { return new String[]{ ChatApp.ObjectCode, Chatter.ObjectCode, Chat.ObjectCode, ChatMessage.ObjectCode, LoginQuery.ObjectCode, ChatHistoryQuery.ObjectCode, ObjectCodes.EventReport // Added in Lesson 13 }; } /** * @return the list of consumed objects - ChatRoom, Chatter, Chat, ChatMessage and ActiveUserQuery */ @Override protected String[] getConsumedObjects() { return new String[]{ ChatRoom.ObjectCode, ChatApp.ObjectCode, Chatter.ObjectCode, Chat.ObjectCode, ChatMessage.ObjectCode, ActiveUserQuery.ObjectCode }; } /** * Add implementation classes to the object factory list of this application. * @param factoryList */ @Override protected void populateObjectFactory(List<Class<? extends DataObject>> factoryList) { super.populateObjectFactory(factoryList); factoryList.add(ChatRoomConsume.class); factoryList.add(ChatApp.class); factoryList.add(ChatterConsume.class); factoryList.add(ChatConsume.class); factoryList.add(ChatMessageImp.class); factoryList.add(LoginQuery.class); factoryList.add(ActiveUserQueryReply.class); factoryList.add(ChatHistoryQueryInquire.class); } private static final String CURRENT_USER_TITLE = "Current user"; private static final String CURRENT_ROOM_TITLE = "Current room"; private static final String CHATTER_TABLE_TITLE = "Room Activity"; private static final String CHATTER_TABLE_TAG = "chatters"; private static final String BAN_BUTTON_LABEL = "Ban Room"; private static final String BAN_BUTTON_TAG = "ban"; private class ChatterTableColumnTitles { static final String NAME = "Chatter name"; static final String UUID = "Application UUID"; static final String JOINED = "Joined since"; static final String LAST = "Last message at"; static final String COUNT = "Message count"; static final String VOLUME = "Message volume"; class SubTitles { static final String COUNT_SINCE = "Since last join"; static final String VOLUME_SINCE = "In bytes since last join"; } } /** * Add a "Current room" title to the "Server information" SpiderAdmin table. * @return the update table structure descriptor. */ @Override public PageInfo.TableInfo getServerInfoTableStructure() { PageInfo.TableInfo tableInfo = super.getServerInfoTableStructure(); return tableInfo. addColumn(CURRENT_USER_TITLE, null, 0, 0). addColumn(CURRENT_ROOM_TITLE, null, 0, 0); } @Override public TableData getServerInfoTableData() { TableData data = super.getServerInfoTableData(); data.getRowData().get(0). addCell(CURRENT_USER_TITLE, myName, 0, null). addCell(CURRENT_ROOM_TITLE, chatter == null ? null : chatter.getRoomName(), 0, null); return data; } /** * Add a "Ban" button and a "Chatters" table to the SpiderAdmin page layout. * @param userID N/A * @return the extended PageInfo object. */ @Override public PageInfo getPageInfo(String userID) { PageInfo.TableInfo table = new PageInfo.TableInfo(CHATTER_TABLE_TITLE, CHATTER_TABLE_TAG, false); table. addColumn(ChatterTableColumnTitles.NAME, null, PageInfo.TableInfo.Style.NONE, PageInfo.TableInfo.Summary.NONE). addColumn(ChatterTableColumnTitles.UUID, null, PageInfo.TableInfo.Style.NONE, PageInfo.TableInfo.Summary.NONE). addColumn(ChatterTableColumnTitles.JOINED, null, PageInfo.TableInfo.Style.NONE, PageInfo.TableInfo.Summary.NONE). addColumn(ChatterTableColumnTitles.LAST, null, PageInfo.TableInfo.Style.NONE, PageInfo.TableInfo.Summary.NONE). addColumn( ChatterTableColumnTitles.COUNT, ChatterTableColumnTitles.SubTitles.COUNT_SINCE, PageInfo.TableInfo.Style.RIGHT, PageInfo.TableInfo.Summary.TOTAL ). addColumn( ChatterTableColumnTitles.VOLUME, ChatterTableColumnTitles.SubTitles.VOLUME_SINCE, PageInfo.TableInfo.Style.RIGHT, PageInfo.TableInfo.Summary.TOTAL ); return super.getPageInfo(userID).addButton(BAN_BUTTON_LABEL, BAN_BUTTON_TAG, true).addTable(table); } /** * Execute the operation of the "Ban" button or return data for the "Chatters" table. * @param serviceTag the tag that identifies the button or the table. * @param userID N/A * @return an OpResults object in the case of a button or a TableData object in the case of a table. */ @Override public Object customAdminService(String serviceTag, String userID) { switch(serviceTag) { case CHATTER_TABLE_TAG: return getChattersTableData(); case BAN_BUTTON_TAG: return banUser(); } return null; } /** * Get the data for the Chatters table * @return a TableData object. */ private TableData getChattersTableData() { TableData data = new TableData(); if (chatter != null) { try { // Get all chatters in this room and sort them by name. ChatRoom room = (ChatRoom)chatter.getParent().getParent(); List<ChatterConsume> users = room.getFilteredChildren(new CatchAllFilter<>(ChatterConsume.class)); Collections.sort(users); // populate the table users.forEach((user) -> { int style = user == chatter ? Style.ALERT : Style.NONE; // Show our line in red String uuid = user.getParent().getObjectID(); // The parent is ChatterApp that is identifed by UUID data.addRow(). addCell( ChatterTableColumnTitles.NAME, user.getName(), style, null ).addCell( ChatterTableColumnTitles.UUID, uuid, style, "xadmin:" + uuid // this links to the SpiderAdmin page of the referred chatting application ).addCell( ChatterTableColumnTitles.JOINED, user.getJoined(), style, null ).addCell( ChatterTableColumnTitles.LAST, user.getLastMessageTime(), style, null ).addCell( ChatterTableColumnTitles.COUNT, user.getMessageCount(), style, null ).addCell( ChatterTableColumnTitles.VOLUME, user.getMessageVolume(), style, null ); }); } catch (NoSuchFieldException | IllegalAccessException ex) { sendExceptionMail(ex, "Exception when collectiing chatters", null, false); } } return data; } /** * Execute the operation of the Ban Room button * @return an OpResults object. */ private OpResults banUser() { // Check if user in a room if (chatter == null) return new OpResults("User is not in a room"); System.out.printf("You are banned from room %sn", chatter.getRoomName()); // Remove the room so that it cannot be joined again. ChatApp chatApp = (ChatApp)chatter.getParent(); chatApp.getParent().remove(); // Make sure the user will not see this room again. chatApp.setBanned(true); chatApp.commit(); // Kick the user out of the room leaveRoom(null); return new OpResults(OpResults.OK); } /** * Process an input line * @param line the line to be broadcast as a chat message. * @return true if processed successfully */ @Override protected boolean processConsoleLine(String line) { try { // If the line starts with '!' this is a command line if (line.startsWith("!")) { String command[] = line.trim().split("s+", 2); switch (command[0].toLowerCase()) { case JOIN: // if room name is provided join the room if (command.length > 1) joinRoom(command[1]); break; case LEAVE: // If the user is in a room, leave it leaveRoom(null); break; case LOGIN: login(); break; case LOGOUT: logout(); break; case REGISTER: register(); break; case HISTORY: if (myName == null) System.out.println("You must log in before requesting history."); else if (!getHistory(command.length < 2 ? null : command[1])) System.out.println("Invalid command argument"); break; } return true; } // If the line starts with '>' split the line on the colon (':') and send the test // after the colon as a private message to the user with the name before the colon. if (line.startsWith(">")) { String cmd[] = line.substring(1).split(":"); if (cmd.length < 2) return true; String destination = findUser(cmd[0]); if (destination == null) return true; // If didn't do yet, create an orphan Chatter object for sending private messages, // then prepare a ChatMessage object for sending private messages if (privateMessage == null) { privateMessage = createTopLevelObject(Chat.class, null); privateMessage.setName(myName); } // Set message and commit to destination privateMessage.setMessage(cmd[1]); privateMessage.setTime(ZDate.now()); privateMessage.commit(destination); return true; } // If this is a normal message, make sure the user is in a room and broadcast the message if (message != null) { message.setMessage(line); message.setTime(ZDate.now()); message.commit(); chatter.setMessageInfo(line); // Update chatter statistics } } catch (Exception ex) { sendExceptionMail(ex, "Exception when processing an input line", line, true); } return true; } public String getMyName() { return myName; } public ZDate getBirthday() { return birthday; } /** * Get user name and password and log in. * @return a PrintStream object. Not needed by the caller but we use it as a trick to save coding. * @throws NoSuchFieldException If LoginQuery does not define ObjectCode static field. * @throws IllegalAccessException If LoginQuery.ObjectCode is not public. */ private PrintStream login() throws NoSuchFieldException, IllegalAccessException { String username; String password; // Check if already logged in if (myName != null) return System.out.printf("You are already logged in as %s. To log in with another name log out first.n", myName); // Create a login query LoginQuery query = createQuery(LoginQuery.class); while(true) { // Get user name System.out.printf("(type Enter to exit login)n"); username = readConsoleLine("User name:"); if (username.isBlank()) return exitLogin(); do { // Get password password = readConsoleLine("Password:"); if (password.isBlank()) return exitLogin(); // Post the login query query.setNewUser(false); query.setName(username); query.setPassword(password); query.post(5 * ZDate.SECOND); // If the query did not expire check login results, if it did, repeat. if (query.waitForReply()) { switch (query.getResult()) { case OK: myName = query.getName(); birthday = query.getBirthday(); return System.out.printf("Logged in successfully as %s.n", myName); case NOT_EXISTS: System.out.printf("User %s does not exist. Please try again.n", username); break; case WRONG_PASSWORD: System.out.printf("Password did not match, please try again.n"); break; } } else return unavailableUserManager(); } while (query.getResult() == LoginQuery.ResultCode.WRONG_PASSWORD); } } /** * Log out if already logged in */ private void logout() { if (myName == null) { System.out.printf("No user is logged in.n"); return; } leaveRoom(null); System.out.printf("%s is logged out.n", myName); myName = null; birthday = null; } private PrintStream register() throws NoSuchFieldException, IllegalAccessException { String username; String password, password2; // Check if already logged in if (myName != null) return System.out.printf("You are already logged in as %s. To register a new user log out first.n", myName); // Create a registration query LoginQuery query = createQuery(LoginQuery.class); while(true) { // Get user name System.out.printf("(type Enter to exit login)n"); username = readConsoleLine("User name:"); if (username.isBlank()) return exitRegistration(); do { // Get password password = readConsoleLine("Password:"); if (password.isBlank()) return exitRegistration(); // Repeat the password password2 = readConsoleLine("Repeat password:"); if (password2.isBlank()) return exitRegistration(); // Check if passwords are equal if (password.equals(password2)) break; System.out.println("Passwords do not match."); } while (true); // Get and parse birth date do { String s = readConsoleLine("Date of birth (yyyy/mm/dd):"); if (s.isBlank()) return exitRegistration(); try { birthday = ZDate.parseTime(s, "yyyy/MM/dd", null); } catch (ParseException ex) { birthday = null; System.out.printf("Invalid date %s. Please try again.n", s); } } while (birthday == null); // Post the registration query query.setNewUser(true); query.setName(username); query.setPassword(password); query.setBirthday(birthday); query.post(5 * ZDate.SECOND); // If the query did not expire check login results, if it did, repeat. if (query.waitForReply()) { switch (query.getResult()) { case OK: myName = username; return System.out.printf("Registered and logged in successfully as %s.n", myName); case EXISTS: System.out.printf("User %s already exista. Please try again with another name.n", username); break; } } else return unavailableUserManager(); } } /** * Print a message when login existed by typing a blank Enter * @return a PrintStream object. Not needed by the caller but we use it as a trick to save coding. */ private PrintStream exitLogin() { return System.out.printf("Login exitedn"); } /** * Print a message when registration existed by typing a blank Enter * @return a PrintStream object. Not needed by the caller but we use it as a trick to save coding. */ private PrintStream exitRegistration() { return System.out.printf("Registration exitedn"); } /** * Print a message when user management is not available * @return a PrintStream object. Not needed by the caller but we use it as a trick to save coding. */ private PrintStream unavailableUserManager() { return System.out.printf("No user management entity is available to handle this requestn"); } /** * Join a room after checking that the room exists, its adult setting matches user configuration, * and the user is not already there. If the user is in another room leave that room first. * @param room the new room name * @throws NoSuchFieldException If any of the created data objects does not define ObjectCode static field. * @throws IllegalAccessException If ObjectCode is not public. */ private synchronized void joinRoom(String room) throws NoSuchFieldException, IllegalAccessException { // User must be logged in if (myName == null) { System.out.printf("You must log in before joining a room.n"); return; } // Check whether room exists and the user is not already in the room ChatRoomConsume chatRoom = getRootObject().getChild(ChatRoomConsume.class, room); if (chatRoom == null) { System.out.printf("Room %s does not existn", room); return; } if (chatter != null && room.equalsIgnoreCase(chatter.getRoomName())) return; // Check if a young chatters is trying to join an adult room if (!chatRoom.canJoin(birthday)) { System.out.printf("You cannot join room %s because it is only for adultsn", room); return; } // Leave current room if any leaveRoom(null); // Join the application to the room ChatApp chatApp = chatRoom.createChild(ChatApp.class, getAppUUID().toString()); // Create a Chatter object for the user that joins the specified room chatter = chatApp.createChild(ChatterConsume.class, myName); chatter.setName(myName); chatter.setBirthday(birthday); chatter.commit(); // let everybody know I joined the room // Prepare a ChatMessage object for sending messages from now on message = chatter.createChild(ChatMessage.class, null); chatter.setJoined(); // Update chatter statistics System.out.printf("Joined room %sn", room); } /** * If the user is in the given room, leave it. * @param room the name of the room to be checked if the user is there. If null, leave the current room without checking */ public synchronized void leaveRoom(String room) { if (isSameRoom(room)) { // Leave the room and let everybody know I did DataObject removed = chatter.remove(); if (removed != null) // can be null if the 'chatter' object has already been removed. removed.commit(); // Disconnect the application from the room and let every application know it did removed = chatter.getParent().remove(); if (removed != null) removed.commit(); System.out.printf("Left room %sn", chatter.getRoomName()); chatter = null; message = null; } } /** * Check if the user is in the same room as the parameter * @param room the name of the room to be checked if the user is there. If null, return true * @return true if the user in 'room' or 'room' is null */ public synchronized boolean isSameRoom(String room) { return chatter != null && (room == null || room.equalsIgnoreCase(chatter.getRoomName())); } /** * Get current room name, if any * @return if the user is currently in a room return the room name, otherwise return null. */ public synchronized String getCurrentRoom() { return chatter == null ? null : chatter.getRoomName(); } /** * Find the user whose name equals the parameter * @param name user name to look for * @return If a Chatter object for this user found then return the object ID of its grandparent, which is the UUID of the * application that the given user runs, otherwise return null. * @throws NoSuchFieldException If any of the referred data objects does not define ObjectCode static field. * @throws IllegalAccessException If ObjectCode is not public. */ private String findUser(String name) throws NoSuchFieldException, IllegalAccessException { FindUserFilter filter = new FindUserFilter(name); Collection<Chatter> users = getRootObject().getFilteredChildren(filter); // If the returned collection is not empty return the object ID (which is a UUID) of the first, // otherwise return null. for (Chatter user : users) { return user.getParent().getObjectID(); } return null; } /** * Execute !history command * @param arg command argument - <n>H or <n>D * @return true if command has been parsed successfully. * @throws NoSuchFieldException If ChatHistoryQuery does not define ObjectCode static field. * @throws IllegalAccessException If ChatHistoryQuery.ObjectCode is not public. */ private boolean getHistory(String arg) throws NoSuchFieldException, IllegalAccessException { // Parse the argument and calculate time ZDate since = null; if (arg != null) { arg = arg.trim().toLowerCase(); int n = Integer.parseInt(arg.substring(0, arg.length() - 1)); if (n <= 0) return false; int unit; switch (arg.substring(arg.length() - 1)) { case "h": unit = ZDate.HOUR; break; case "d": unit = ZDate.DAY; break; default: return false; } since = ZDate.now().add(-n * unit); } // create a query and post it ChatHistoryQuery query = createQuery(ChatHistoryQuery.class); query.setUsername(myName); query.setSince(since); query.post(5 * ZDate.SECOND); return true; } }
The SpiderAdmin customization code starts at line 152, where string constants that are used for titles and labels are declared.
The method getServerInfoTableStructure() is overridden (line 176) to add the two new columns to the layout of the Server Information table. The contents of the new cells (identified by column titles) are provided in getServerInfoTableData() (line 184).
The method getPageInfo() is overridden (line 198) to add the Ban Room button and the Room Activity table to the layout of the application’s page.
The last method that we need to override is customAdminService() (line 227), where we check
the serviceTag
argument (taken from
the values provided by getPageInfo()
)
and switch accordingly to either executing the button function or providing the
data for the new table.
The content of the new table is provided by getChattersTableData()
(line 241). The data is generated by applying the getFilteredChildren() method with a CatchAllFilter on the ChatRoom
object that represents the current room to retrieve a list of all its chatters and then sort the list by user name (we will see below how Chatter
is modified in order to enable the sort). We then use each ChatterConsume
object of the sorted list to fill in the content of one table row. The code is pretty straightforward but there are few points that deserve special attention:
First, we want to display the table row that represents the
current user in red. This is achieved by setting
the style
argument of addCell() to Style.ALERT.
Secondly, note that some data cells contain time values. The
data for these cells is provided as Date
objects. SpiderAdmin is smart enough to display these values in the locale of
the browser.
Lastly, note that when inserting cell data in the Application UUID column we provide a uri
argument that consists of the UUID
preceded by "xadmin:"
. This
trick causes SpiderAdmin to show the UUID value as a link to the SpiderAdmin
page of the linked application!
The Ban Room
button is handled in banUser()
(line
298). The method prints a message, sets the banned
field of the related ChatApp
object
to true
, commits the object and then
calls leaveRoom()
to leave the room.
The other modifications to ChatMain
are the call to ChatterConsume.setMessageInfo()
(line 385) when the user types a message and to ChatterConsume.setJoined()
(line 603) when the user joins a room.
We will see these methods in a moment.
As we have just mentioned, the data for the Room Activity table is taken from ChatterConsume
objects. Here is the
class:
package org.spiderwiz.tutorial.lesson18.chat; import org.spiderwiz.tutorial.objectLib.Chatter; import org.spiderwiz.zutils.ZDate; /** * Consumer implementation of Chatter data object. Notify when a chatter enters or leaves my room. */ public class ChatterConsume extends Chatter implements Comparable<ChatterConsume> { private ZDate joined = null; private ZDate lastMessageTime = null; private int messageCount = 0; private int messageVolume = 0; /** * Notify when a chatter enters my room. */ @Override protected void onNew() { if (!isStealth() && ChatMain.getInstance().isSameRoom(getRoomName()) && getName() != null) System.out.printf("%s entered the roomn", getName()); setJoined(); } /** * Notify when a chatter leaves my room. If the chatter is myself leave the room gracefully. * @return true to confirm the removal. */ @Override protected boolean onRemoval() { if (ChatMain.getInstance().getAppUUID().toString().equals(getParent().getObjectID())) ChatMain.getInstance().leaveRoom(getRoomName()); else if (!isStealth() && ChatMain.getInstance().isSameRoom(getRoomName()) && getName() != null) System.out.printf("%s left the roomn", getName()); return true; } /** * Utility function to get the room name, which is the ID of the grand parent of the current object. * @return the room name */ String getRoomName() { return getParent().getParent().getObjectID(); } /** * @return the time the chatter joined the room. */ public synchronized ZDate getJoined() { return joined; } /** * Sets the "joined" time to now. */ public void setJoined() { this.joined = ZDate.now(); } /** * @return the time of the last message of this chatter in the room. */ public synchronized ZDate getLastMessageTime() { return lastMessageTime; } /** * @return the number of messages that the sender sent in the room since joining. */ public synchronized int getMessageCount() { return messageCount; } /** * * @return the volume of the messages in bytes that the sender sent in the room since joining. */ public synchronized int getMessageVolume() { return messageVolume; } /** * Update statistics when a message from this chatter arrives in the room. * @param message */ public synchronized void setMessageInfo(String message) { lastMessageTime = ZDate.now(); ++messageCount; messageVolume += message.length(); } /** * Compare objects by comparing the name property. * @param o the object to compare to. * @return negative value if this object is the lower, zero if they are equal and a positive value if this object is the higher. */ @Override public int compareTo(ChatterConsume o) { String myName = getName(); String herName = o.getName(); return myName == null ? herName == null ? 0 : -1 : herName == null ? 1 : myName.compareTo(herName); } }
The first thing that you can notice is that the class now
implements Comparable, so that lists of this class are
sortable as we saw above. We also add four properties – joined
,
lastMessageTime
, messageCount
and messageVolume
that hold the information that we need for the Room Activity table. These properties have getters
in lines 49, 63, 70 and 78 correspondingly.
The method onNew() is called when a chatter joins the
current room. In this method we add a call to setJoined()
(line 22) that saves the join time in the joined
property. We also add the setMessageInfo()
method that is called
when a message is typed in the room. The message is provided as the method
argument and is used to update object properties as needed.
The method compareTo() is implemented (line 98) to compare
class objects by comparing their name
properties.
The other class that we need to modify is ChatMessageImp
:
package org.spiderwiz.tutorial.lesson18.chat; import java.util.Map; import java.util.UUID; import org.spiderwiz.tutorial.objectLib.ChatApp; import org.spiderwiz.tutorial.objectLib.ChatMessage; import org.spiderwiz.tutorial.objectLib.ChatRoom; /** * Consumer implementation of the ChatMessage class */ public class ChatMessageImp extends ChatMessage { /** * Called when a chat message is received. Check if the message has been sent in the room we are in, * then print the sender name and the message. Also update chatter's statistics. * @return true to indicate that the event has been handled. */ @Override protected boolean onEvent() { if (ChatMain.getInstance().isSameRoom(getParent().getParent().getParent().getObjectID())) { ChatterConsume chatter = (ChatterConsume)getParent(); String name = chatter.getName(); if (name == null) name = chatter.getObjectID(); System.out.printf("%1$s: %2$sn", name, getMessage()); chatter.setMessageInfo(getMessage()); } return true; } /** * Restrict message sending to: * 1. applications that are not the current application. * 2. applications that are on the same chat room as the current user, or: * 3. applications whose 'appParams' contains the mapping 'role'->'admin' * @param appUUID destination application UUID * @param appName destination application name * @param userID destination user ID * @param remoteAddress remote network address * @param appParams application parameters of the destination application * @return true if the object should be sent to this destination */ @Override protected boolean filterDestination(UUID appUUID, String appName, String userID, String remoteAddress, Map<String, String> appParams ) { return !ChatMain.getInstance().getAppUUID().equals(appUUID) && ( isMemberOfMyRoom(appUUID) || appParams != null && "admin".equals(appParams.get("role")) ); } /** * Check if the user running the application whose UUID is given is on the same room as this message is sent to. * @param appUUID Application UUID to check * @return true if the application is in the same room as us. */ private boolean isMemberOfMyRoom(UUID appUUID) { try { // The room is the great-grandparent of the message ChatRoom chatRoom = (ChatRoom)getParent().getParent().getParent(); return chatRoom.getChild(ChatApp.class, appUUID.toString()) != null; } catch (NoSuchFieldException | IllegalAccessException ex) { // Arriving here if a ChatApp does not contain ObjectCode static field or the field is not public. // In this case, send a command exception message. ChatMain.getInstance().sendExceptionMail(ex, "Cannot instantiate ChatApp", null, false); return false; } } }
We add code in onEvent() (line 19) that updates the parent ChatterConsume
object when a message is
received.
We are almost done but we have a little problem. The changes
that we made to the Chat application basically did the job. I.e. when the
administrator clicks the Ban Room
button on the application’s page the user is kicked out of the room and the ChatRoom
object is deleted so that the
user cannot join the room again. But what if the application restarts or
reconnects to the network? In these cases the application, as a consumer of ChatRoom
, requests a reset for this
object type and gets all available rooms including the banned one.
Here comes to rescue the banned
property that we added to ChatApp
. We
will show its use with the Room Manager
application which was introduced in Lesson 5.
We will use it to restrict the routing of ChatRoom
objects so that applications that are banned from a room will not get it. Here
is RoomManagerMain
:
package org.spiderwiz.tutorial.lesson18.roomManager; import java.util.List; import org.spiderwiz.core.DataObject; import org.spiderwiz.tutorial.objectLib.ChatApp; import org.spiderwiz.tutorial.objectLib.ChatRoom; import org.spiderwiz.tutorial.objectLib.ConsoleMain; /** * Provides the entry point of the application. Initializes and executes the Spiderwiz framework. */ public class RoomManagerMain extends ConsoleMain { private static final String ROOT_DIRECTORY = ""; private static final String CONF_FILENAME = "room-manager.conf"; private static final String APP_NAME = "Room Manager"; private static final String APP_VERSION = "Z18.01"; // Version Z18.01: Lesson 18 version private static final String CREATE = "create"; // create chat room command private static final String DELETE = "delete"; // delete chat room command private static final String MODIFY = "modify"; // modify chat room command private static final String RENAME = "rename"; // rename chat room command /** * Class constructor with constant parameters. */ public RoomManagerMain() { super(ROOT_DIRECTORY, CONF_FILENAME, APP_NAME, APP_VERSION); } /** * Application entry point. Instantiate the class and initialize the instance. * * @param args the command line arguments. Not used in this application. */ public static void main(String[] args) { new RoomManagerMain().init(); } /** * Print out usage instructions at start up * @return true */ @Override protected boolean preStart() { System.out.printf( "To create a new room: type 'create <room name>'.n" + "Append a plus sign (+) to the name if you want to create an adult only room.n" + "To delete a room type 'delete <room name>'.n" + "To modify the adult categorization of a room:n" + "Type 'modify <room name>+' if you want to make it an adult room.n" + "Type 'modify <room name>' if you want to remove the adult categorization.n" + "To rename a room type 'rename <room name>=<new name>'.n" + "To exit type 'exit'.n" ); return true; } /** * @return the list of produced objects, Chat is the only one. */ @Override protected String[] getProducedObjects() { return new String[]{ ChatRoom.ObjectCode }; } /** * @return the list of consumed objects, we need ChatApp for banning certain applications from certain rooms. */ @Override protected String[] getConsumedObjects() { return new String[]{ ChatApp.ObjectCode }; } /** * Add ChatRoom class to the object factory list of this application. * @param factoryList */ @Override protected void populateObjectFactory(List<Class<? extends DataObject>> factoryList) { super.populateObjectFactory(factoryList); factoryList.add(ChatRoomProduce.class); factoryList.add(ChatAppConsume.class); } /** * Process an input line * @param line An input line that contains a room management command * @return true if processed successfully */ @Override protected boolean processConsoleLine(String line) { try { // Trim line and split to command / parameter. // Then check if there is a parameter and whether it contains the adult symbol. // If there is not parameter, ignore the command. String command[] = line.trim().split("s+", 2); if (command.length < 2) return true; String name[] = command[1].split("+", -1); boolean adult = name.length > 1; // now split for 'rename' command name = name[0].split("="); String roomName = name[0]; // If exists, get the ChatRoom object ChatRoom room = getRootObject().getChild(ChatRoom.class, roomName); // Switch by command and process it switch (command[0].toLowerCase()) { case CREATE: // Create a new room if does not exist (ignore if it does) if (room == null) { room = createTopLevelObject(ChatRoom.class, roomName); room.setAdult(adult); room.commit(); System.out.printf("Room %1$s(%2$s) createdn", roomName, adult ? "adult" : "unrestricted"); } break; case DELETE: // Delete the room if exists if (room != null) { room.remove(); room.commit(); System.out.printf("Room %1$s deletedn", roomName); } break; case MODIFY: // Modify the 'adult' field if the room exits if (room != null) { room.setAdult(adult); room.commit(); System.out.printf("Room %1$s set to %2$sn", roomName, adult ? "adult" : "unrestricted"); } break; case RENAME: // Rename the room if exists. The rename command is 'rename <old name>=<new name>' if (room != null && name.length > 1) { String newName = name[1]; if (!newName.equalsIgnoreCase(roomName)) { DataObject renamed = room.rename(newName); if (renamed == null) System.out.printf("Cannot rename to already existing room name %sn", newName); else { renamed.commit(); System.out.printf("Room %1$s was renamed %2$sn", roomName, newName); } } } } return true; } catch (NoSuchFieldException | IllegalAccessException ex) { // Theoretically arriving here if any data object does not contain ObjectCode static field or the field is not public. // In this case, send a command exception message. sendExceptionMail(ex, "Cannot instantiate a data object class", null, false); return false; } } }
There are two changes here. The application is now a
consumer of ChatApp
(line 74) so that
it is notified when an application instance is banned from a room. Additionally,
two implementation classes – ChatRoomProduce
and ChatAppConsume
have been added to
the package and are registered in populateObjectFactory() (line 83).
This is what we do in ChatRoomProduce
:
package org.spiderwiz.tutorial.lesson18.roomManager; import java.util.Map; import java.util.UUID; import org.spiderwiz.tutorial.objectLib.ChatApp; import org.spiderwiz.tutorial.objectLib.ChatRoom; /** * Extends ChatRoom to make sure the room is not published to banned users. */ public class ChatRoomProduce extends ChatRoom { /** * Filter out banned users. * @param appUUID application UUID. * @param appName application name. * @param userID the user ID attached to the network channel through which the destination application is connected to the * current application. * @param remoteAddress remote address of the destination application. * @param appParams application parameter map as set by {@link org.spiderwiz.core.Main#getAppParams()} * method of the destination application. May be null if the destination application did not define any * parameters. * @return true to approve distribution to this application, false to deny it. */ @Override protected boolean filterDestination(UUID appUUID, String appName, String userID, String remoteAddress, Map<String, String> appParams ) { try { ChatApp chatApp = getChild(ChatApp.class, appUUID.toString()); return chatApp == null || !chatApp.isBanned(); } catch (NoSuchFieldException | IllegalAccessException ex) { RoomManagerMain.getInstance().sendExceptionMail(ex, "Cannot instantiate ChatApp", null, false); return false; } } }
The method filterDestination() is overridden (line 26) to
filter out banned application instances from receiving the banned rooms.
Simple, no? Wait a moment! How do we know which instances are banned? From the banned
property of the corresponding ChatApp
object, right? But don’t we kick
out a user from a room by deleting the associated ChatApp
object? If so, how can we get the banned
property of a deleted object?
Well, the answer is in ChatAppConsume
:
package org.spiderwiz.tutorial.lesson18.roomManager; import org.spiderwiz.tutorial.objectLib.ChatApp; /** * Implements ChatApp to support persistent user ban */ public class ChatAppConsume extends ChatApp{ /** * If the application is marked as "banned" prevent object removal so that the room (parent of this object) will not be sent * to the application when it requests a reset. * @return true if not banned, false if it is. */ @Override protected boolean onRemoval() { return !isBanned(); } }
All we need to do is to override onRemoval() to return false
if the banned
property is true
. That causes the
framework to locally ignore the removal and keep the object in its space, so
that ChatRoomProduce
can filter it
out. Mission done!
Here is a sample of the resulted Chat application page on SpiderAdmin:
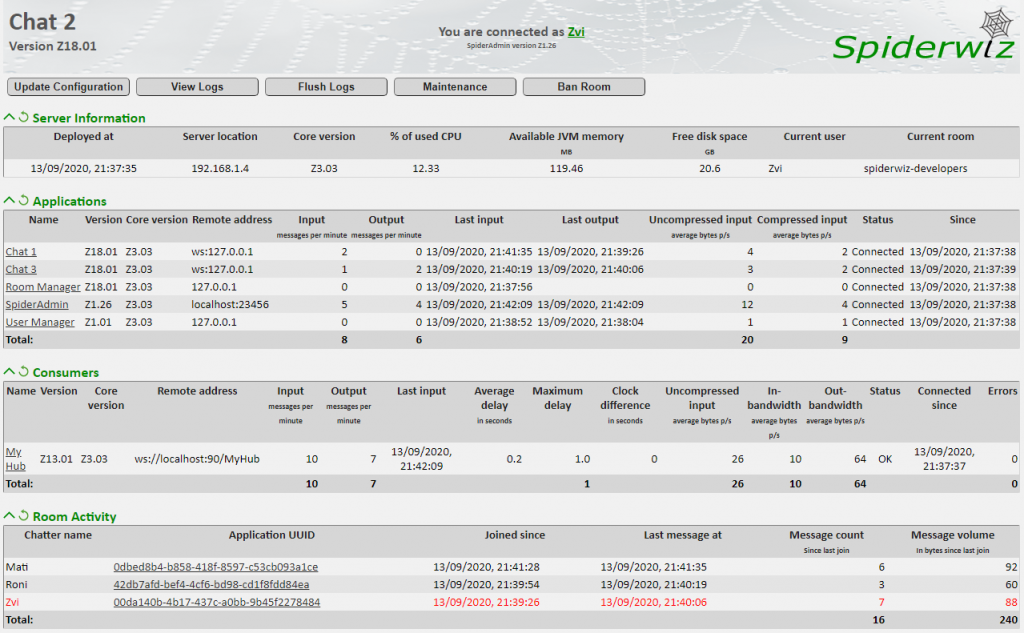
With this cool stuff we are arriving to the end of this tutorial. End but not completed. There are more readings and activities that we can recommend for those who want to become real Spiderwiz experts. More about it here.